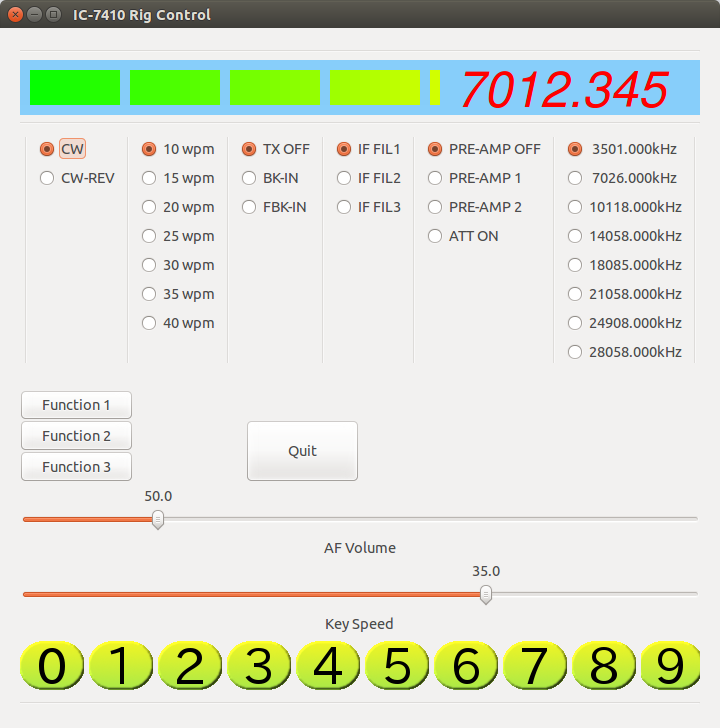
It does not look very cool, but is quite acceptable. Do you agree?
struct radio_button {
int nbutton;
char name[32][128];
};
int ngroup = 6;
struct radio_button my_radio_buttons[128] = {
{2, {"CW", "CW-REV"} },
{7, {"10 wpm", "15 wpm", "20 wpm", "25 wpm", "30 wpm", "35 wpm", "40 wpm"} },
{3, {"TX OFF", "BK-IN" , "FBK-IN"} },
{3, {"IF FIL1", "IF FIL2" , "IF FIL3"} },
{4, {"PRE-AMP OFF", "PRE-AMP 1" , "PRE-AMP 2", "ATT ON"} },
{8, {" 3501.000kHz", " 7026.000kHz", "10118.000kHz", "14058.000kHz",
"18085.000kHz", "21058.000kHz", "24908.000kHz", "28058.000kHz"} },
};
This is to define radio buttons.
GtkWidget *hbox_radio, *vbox_radio;
hbox_radio = gtk_hbox_new(FALSE, 5);
for(int j=0;j<ngroup;j++) {
vbox_radio = gtk_vbox_new(FALSE, 5);
for(int i=0;i<my_radio_buttons[j].nbutton;i++) {
if(i == 0) {
button = gtk_radio_button_new_with_label(NULL,
my_radio_buttons[j].name[i]);
} else {
button = gtk_radio_button_new_with_label(gtk_radio_button_group (GTK_RADIO_BUTTON (button)),
my_radio_buttons[j].name[i]);
}
gtk_signal_connect (GTK_OBJECT (button), "toggled", GTK_SIGNAL_FUNC (callback),
(gpointer) my_radio_buttons[j].name[i]);
gtk_box_pack_start (GTK_BOX (vbox_radio), button, FALSE, FALSE, 0);
gtk_widget_show (button);
}
gtk_box_pack_start (GTK_BOX (hbox_radio), vbox_radio, FALSE, FALSE, 0);
}
And this is for drawing the buttons. The names of the buttons must be unique, because they all connected to the same callback routine.